Python自动化炒股:利用CatBoost和XGBoost进行股票市场预测的实战案例
Python自动化炒股:利用CatBoost和XGBoost进行股票市场预测的实战案例
引言
在当今的金融市场中,自动化交易已经成为一种趋势。Python,以其强大的数据处理能力和丰富的库支持,成为了自动化交易的首选工具。本文将带你了解如何使用Python中的CatBoost和XGBoost算法来进行股票市场预测,帮助你在股市中把握先机。
什么是CatBoost和XGBoost?
CatBoost和XGBoost都是基于梯度提升决策树(Gradient Boosting Decision Trees, GBDT)的算法。它们在处理分类和回归问题上表现出色,尤其是在金融领域,能够处理大量特征和非线性关系。
- XGBoost:eXtreme Gradient Boosting,它优化了梯度提升算法,提高了计算效率,并且支持并行处理。
- CatBoost:Categorical Boosting,它专门处理分类变量,无需手动编码,能够自动处理类别特征。
环境准备
在开始之前,确保你的Python环境中安装了以下库:
!pip install numpy pandas scikit-learn xgboost catboost matplotlib
数据准备
我们将使用一个公开的股票市场数据集来进行预测。这里我们假设你已经有了一个CSV文件,包含了股票的历史价格和交易量等信息。
import pandas as pd
# 加载数据
data = pd.read_csv('stock_data.csv')
print(data.head())
数据预处理
在进行模型训练之前,我们需要对数据进行预处理,包括缺失值处理、特征编码等。
# 缺失值处理
data.fillna(method='ffill', inplace=True)
# 特征编码
data['Date'] = pd.to_datetime(data['Date'])
data['Day_of_Week'] = data['Date'].dt.dayofweek
data['Month'] = data['Date'].dt.month
特征选择
选择对预测有帮助的特征是提高模型性能的关键。
# 选择特征
features = ['Open', 'High', 'Low', 'Volume', 'Day_of_Week', 'Month']
X = data[features]
y = data['Close'].shift(-1) # 预测下一个交易日的收盘价
模型训练
我们将分别使用XGBoost和CatBoost来训练模型。
XGBoost模型
import xgboost as xgb
# 划分数据集
X_trAIn, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 训练XGBoost模型
xgb_model = xgb.XGBRegressor(objective='reg:squarederror', n_estimators=100)
xgb_model.fit(X_train, y_train)
CatBoost模型
from catboost import CatBoostRegressor
# 训练CatBoost模型
cat_model = CatBoostRegressor(iterations=100, depth=3, learning_rate=0.1)
cat_model.fit(X_train, y_train, cat_features=['Day_of_Week', 'Month'])
模型评估
我们使用均方误差(MSE)来评估模型的性能。
from sklearn.metrics import mean_squared_error
# XGBoost模型评估
xgb_pred = xgb_model.predict(X_test)
xgb_mse = mean_squared_error(y_test, xgb_pred)
print(f"XGBoost MSE: {xgb_mse}")
# CatBoost模型评估
cat_pred = cat_model.predict(X_test)
cat_mse = mean_squared_error(y_test, cat_pred)
print(f"CatBoost MSE: {cat_mse}")
结果对比
通过比较两个模型的MSE,我们可以确定哪个模型更适合我们的数据。
# 比较模型性能
if xgb_mse < cat_mse:
print("XGBoost模型表现更好。")
else:
print("CatBoost模型表现更好。")
预测与交易
最后,我们可以使用表现更好的模型来进行股票市场的预测,并据此制定交易策略。
# 使用CatBoost进行预测
final_pred = cat_model.predict(X_test)
# 绘制预测结果
import matplotlib.pyplot as plt
plt.figure(figsize=(10, 6))
plt.plot(y_test.index, y_test, label='Actual Price')
plt.plot(y_test.index, final_pred, label='Predicted Price')
plt.title('Stock Price Prediction')
plt.xlabel('Date')
plt.ylabel('Price')
plt.legend()
plt.show()
结语
通过本文的实战案例,你已经学会了如何使用CatBoost和XGBoost来进行股票市场预测。记住,模型的选择
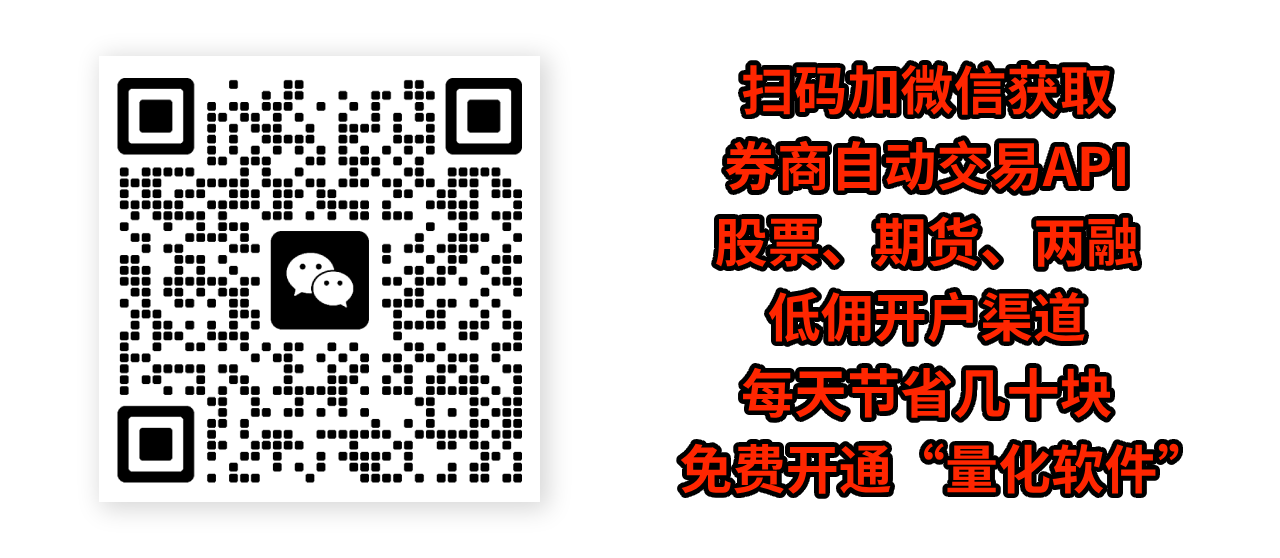
5.16 绩效评估的常见风险管理技术
« 上一篇
2024-08-08
如何利用Python进行股票市场的3D打印分析?
下一篇 »
2024-08-09