Python自动化炒股:基于自然语言处理的股票新闻情感分析模型开发与优化的详细指南

Python自动化炒股:基于自然语言处理的股票新闻情感分析模型开发与优化的详细指南
在当今这个信息爆炸的时代,股票市场受到各种新闻和社交媒体的影响越来越大。投资者们越来越依赖于自动化工具来分析市场情绪,以做出更明智的投资决策。本文将带你深入了解如何使用Python和自然语言处理(NLP)技术来开发一个股票新闻情感分析模型,帮助你在股市中抓住机遇。
一、了解情感分析
情感分析,也称为意见挖掘,是一种计算机系统,能够自动检测、提取、量化和研究人们的情感倾向。在股票新闻分析中,我们的目标是识别新闻文本中的情感倾向,以预测市场对特定股票的反应。
二、准备工作
在开始编码之前,我们需要准备一些工具和数据:
- Python环境:确保你的计算机上安装了Python。
- NLP库:我们将使用
nltk
和textblob
进行文本预处理和情感分析。 - 数据集:你可以从网上找到公开的股票新闻数据集,或者使用API获取实时新闻。
三、环境搭建
首先,我们需要安装必要的Python库:
pip install nltk textblob
四、数据预处理
在进行情感分析之前,我们需要对文本数据进行预处理。这包括去除停用词、标点符号、数字等。
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
# 下载NLTK的停用词集
nltk.download('stopwords')
nltk.download('punkt')
def preprocess_text(text):
# 将文本转换为小写
text = text.lower()
# 分词
words = word_tokenize(text)
# 去除停用词和非字母字符
words = [word for word in words if word.isalpha() and not word in stopwords.words('english')]
return ' '.join(words)
五、情感分析
我们将使用TextBlob
进行简单的情感分析。TextBlob
提供了一个sentiment
属性,其中包含了polarity
和subjectivity
两个值,polarity
的范围是-1到1,表示情感的正负。
from textblob import TextBlob
def analyze_sentiment(text):
blob = TextBlob(text)
return blob.sentiment.polarity
六、模型开发
现在,我们将开发一个简单的模型,该模型将根据新闻的情感分析结果来预测股票价格的变动。
def predict_stock_price(news_text, current_price):
# 预处理新闻文本
processed_text = preprocess_text(news_text)
# 进行情感分析
sentiment = analyze_sentiment(processed_text)
# 根据情感分析结果预测股票价格变动
if sentiment > 0.1: # 假设正向情感导致价格上涨
return current_price * 1.05
elif sentiment < -0.1: # 假设负向情感导致价格下跌
return current_price * 0.95
else:
return current_price
七、优化模型
为了提高模型的准确性,我们可以考虑使用更复杂的NLP技术和机器学习算法。例如,使用scikit-learn
库中的逻辑回归模型。
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.linear_model import LogisticRegression
# 假设我们有一个标签化的数据集
news_texts = ["Stock A is doing great!", "Stock B is in trouble.", ...]
labels = [1, -1, ...] # 1表示正面新闻,-1表示负面新闻
# 文本向量化
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(news_texts)
# 训练模型
model = LogisticRegression()
model.fit(X, labels)
# 预测函数
def predict_stock_price_with_model(news_text):
X_new = vectorizer.transform([news_text])
sentiment = model.predict(X_new)
return predict_stock_price(news_text, current_price) if sentiment[0] == 1 else current_price * 0.95
八、实时数据集成
为了使模型能够实时分析最新的股票新闻,我们可以集成一个新闻API,如NewsAPI
。
import requests
def get_latest_news(api_key, query):
url = f"https://newsapi.org/v2/everything?q={query}&apiKey={api_key}"
response = requests.get(url)
articles = response.json().get('articles', [])
return articles
# 使用API获取最新的股票新闻
api_key = 'your_api_key_here'
latest_news = get_latest_news(api_key, 'stock market')
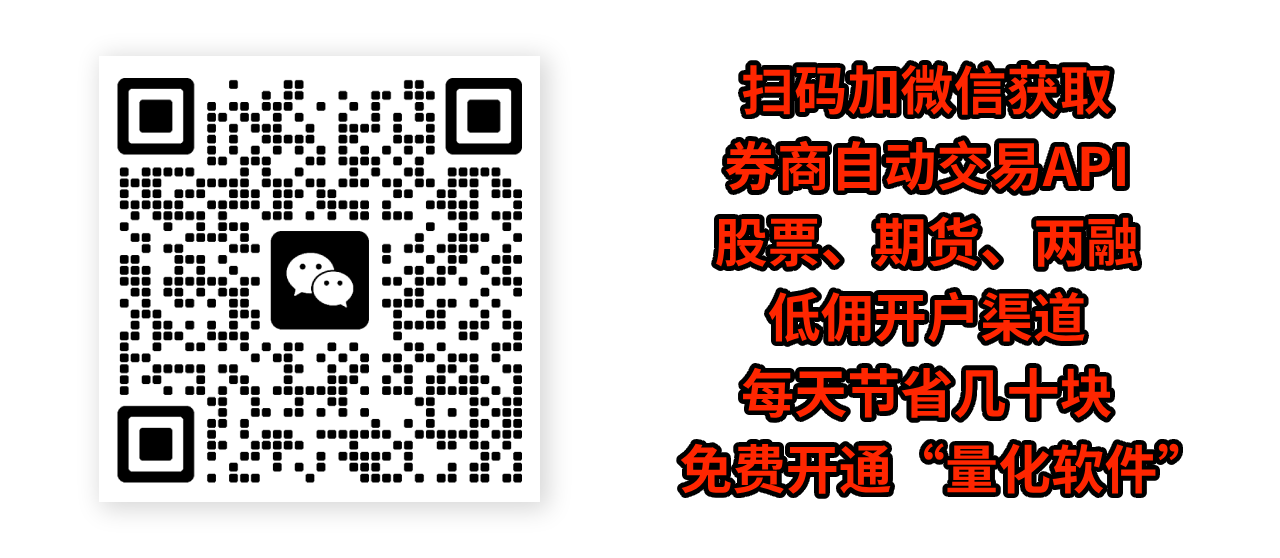
8.22 常见人工智能问题的解决方案
« 上一篇
2025-02-22
Python自动化炒股:使用Plotly Express和Dash进行股票数据可视化的实战案例
下一篇 »
2025-02-24