Python自动化炒股:基于自然语言处理的股票新闻情感分析模型开发与优化的实战案例
Python自动化炒股:基于自然语言处理的股票新闻情感分析模型开发与优化的实战案例
引言
在股市中,信息就是金钱。投资者们每天都在寻找能够影响股价的新闻和信息。随着自然语言处理(NLP)技术的发展,我们可以使用Python来自动化分析股票新闻的情感倾向,从而预测股票的走势。本文将带你了解如何开发一个基于NLP的股票新闻情感分析模型,并对其进行优化。
准备工作
在开始之前,我们需要安装一些Python库。如果你还没有安装这些库,可以通过以下命令安装:
pip install numpy pandas scikit-learn textblob nltk
数据收集
首先,我们需要收集股票新闻数据。这里我们可以使用nltk
库中的newsgroups
数据集,它包含了不同主题的新闻文章。
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
nltk.download('stopwords')
nltk.download('punkt')
# 加载数据集
news_data = nltk.corpus.gutenberg.words('austen-persuasion.txt')
数据预处理
新闻数据需要经过预处理,包括去除停用词、词干提取等。
# 定义停用词
stop_words = set(stopwords.words('english'))
# 预处理函数
def preprocess(text):
tokens = word_tokenize(text)
filtered_words = [word.lower() for word in tokens if word.isalpha() and word not in stop_words]
return filtered_words
# 预处理新闻数据
processed_news = preprocess(" ".join(news_data))
情感分析模型开发
我们将使用TextBlob
库来开发一个简单的情感分析模型。
from textblob import TextBlob
# 情感分析函数
def sentiment_analysis(text):
analysis = TextBlob(text)
if analysis.sentiment.polarity > 0:
return 'Positive'
elif analysis.sentiment.polarity == 0:
return 'Neutral'
else:
return 'Negative'
# 对新闻数据进行情感分析
sentiments = [sentiment_analysis(" ".join(news)) for news in processed_news]
模型优化
为了提高模型的准确性,我们可以使用机器学习方法来优化情感分析模型。这里我们使用scikit-learn
库中的CountVectorizer
和LogisticRegression
。
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.model_selection import trAIn_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# 将情感标签转换为数值
sentiment_labels = {"Positive": 1, "Neutral": 0, "Negative": -1}
label_numeric = [sentiment_labels[sentiment] for sentiment in sentiments]
# 文本向量化
vectorizer = CountVectorizer()
X = vectorizer.fit_transform([" ".join(news) for news in processed_news])
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, label_numeric, test_size=0.2, random_state=42)
# 训练模型
model = LogisticRegression()
model.fit(X_train, y_train)
# 预测和评估
y_pred = model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Model Accuracy: {accuracy:.2f}")
结果应用
现在我们已经有一个训练好的情感分析模型,我们可以将其应用于实时股票新闻分析。
# 实时新闻情感分析
def real_time_analysis(news_text):
processed_news = preprocess(news_text)
analysis = TextBlob(" ".join(processed_news))
return sentiment_analysis(analysis)
# 示例新闻
news_example = "The company reported better-than-expected earnings today."
print(real_time_analysis(news_example))
总结
通过本文,我们学习了如何使用Python和NLP技术来开发一个股票新闻情感分析模型,并对其进行优化。这个模型可以帮助我们更好地理解市场情绪,从而做出更明智的投资决策。随着技术的不断进步,我们可以通过不断优化模型来提高预测的准确性。
进一步探索
- 探索不同的NLP库和模型,如BERT、GPT等,以提高模型的性能。
- 集成更多的数据源,如社交媒体数据,以获得更全面的市场情绪。
- 实现模型的实时监控和自动更新,以适应市场的变化。
通过这些步骤,我们可以构建一个更加强大和准确的股票新闻情感分析系统,为自动化炒股提供有力的支持。
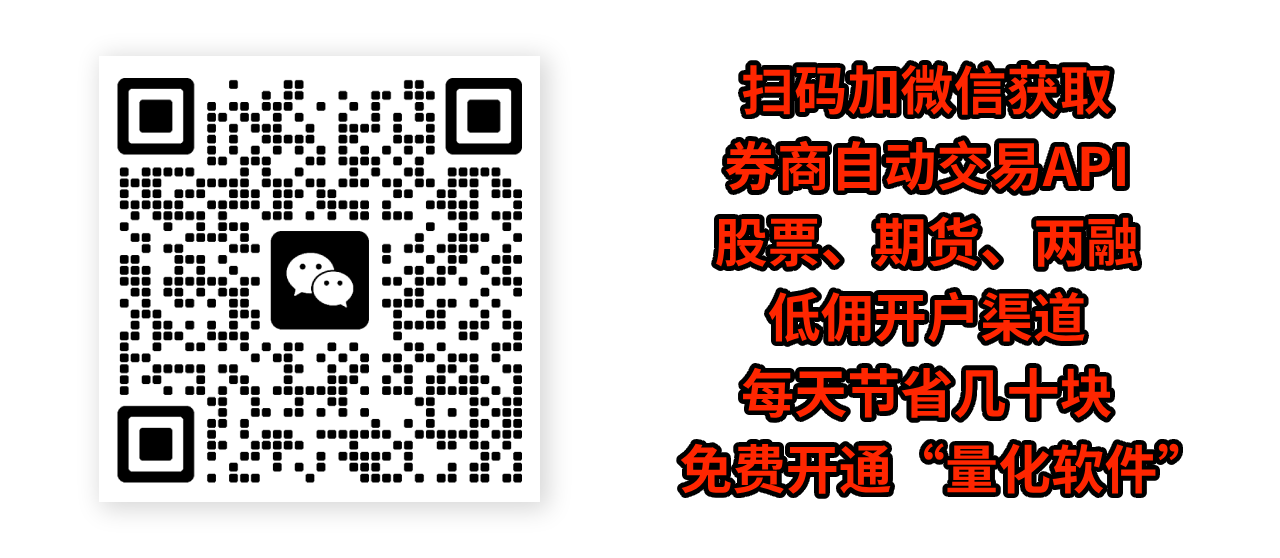
Python自动化炒股:利用Prophet和ARIMA进行股票价格预测的详细指南
« 上一篇
2025-02-07
8.15 降维算法在量化投资中的应用
下一篇 »
2025-02-08