Python自动化炒股:基于强化学习的股票交易策略优化与实现的详细指南

Python自动化炒股:基于强化学习的股票交易策略优化与实现的详细指南
在金融市场的海洋中,炒股就像航海,需要智慧和勇气。随着技术的发展,Python作为一种强大的编程语言,已经成为许多交易者自动化炒股的首选工具。今天,我们将探索如何使用Python和强化学习来优化股票交易策略。这篇文章将带你走进自动化炒股的世界,让你的交易策略更加智能化。
什么是强化学习?
强化学习是一种机器学习方法,它通过与环境的交互来学习如何做出决策。在股票交易中,环境是股市,而决策则是买入或卖出股票。强化学习的目标是最大化累积奖励,这在股票交易中可以转化为最大化利润。
为什么选择强化学习?
- 适应性:强化学习模型能够适应市场的变化,自动调整策略。
- 自主性:一旦训练完成,模型可以独立做出交易决策,无需人工干预。
- 优化:通过不断学习,模型可以优化其策略,以获得更高的回报。
环境设置
在开始之前,我们需要安装一些必要的Python库:
!pip install numpy pandas matplotlib gym
构建股票交易环境
我们将使用OpenAI Gym库来构建一个简单的股票交易环境。这个环境将模拟股票价格的变动,并根据交易决策给予奖励。
import gym
from gym import spaces
import numpy as np
import pandas as pd
class StockTradingEnv(gym.Env):
metadata = {'render.modes': ['console']}
def __init__(self, stock_price):
super(StockTradingEnv, self).__init__()
self.stock_price = stock_price
self.action_space = spaces.Discrete(3) # 0: Hold, 1: Buy, 2: Sell
self.observation_space = spaces.Box(low=0, high=1, shape=(1,), dtype=np.float32)
self.state = 0
self.done = False
def step(self, action):
if self.done:
raise Exception("Episode is done. Reset the environment.")
reward = 0
self.state = (self.state + 1) % len(self.stock_price)
current_price = self.stock_price[self.state]
if action == 1: # Buy
self.portfolio['holdings'] += 1
self.portfolio['cash'] -= current_price
elif action == 2 and self.portfolio['holdings'] > 0: # Sell
self.portfolio['holdings'] -= 1
self.portfolio['cash'] += current_price
self.portfolio['total'] = self.portfolio['cash'] + self.portfolio['holdings'] * current_price
reward = self.portfolio['total'] - self.initial_total
if self.state == len(self.stock_price) - 1:
self.done = True
return np.array([current_price]), reward, self.done, {}
def reset(self):
self.state = 0
self.portfolio = {'cash': 10000, 'holdings': 0, 'total': 10000}
self.initial_total = self.portfolio['total']
self.done = False
return np.array([self.stock_price[self.state]])
def render(self, mode='console', close=False):
if close:
return
print(f"Day {self.state + 1}: Cash = {self.portfolio['cash']}, Holdings = {self.portfolio['holdings']}")
# Example usage
stock_data = pd.read_csv('stock_data.csv')
env = StockTradingEnv(stock_data['Close'].values)
env.reset()
env.render()
强化学习模型
我们将使用Q-learning,这是一种简单的强化学习算法,来训练我们的交易策略。
import random
class QLearningAgent:
def __init__(self, actions, learning_rate, reward_decay, e_greedy):
self.actions = actions
self.lr = learning_rate
self.gamma = reward_decay
self.epsilon = e_greedy
self.q_table = pd.DataFrame(columns=self.actions, dtype=np.float64)
def choose_action(self, observation):
if np.random.uniform() < self.epsilon:
state_action = self.q_table.loc[observation, :]
action = np.random.choice(state_action[state_action == np.max(state_action)].index)
else:
action = np.random.choice(self.actions)
return action
def learn(self, s, a, r, s_):
q_predict = self.q_table.loc[s, a]
if s_ != 'terminal':
q_target = np.max(self.q_table.loc[s_, :])
else:
q_target = 0
q_target = (self.lr * (
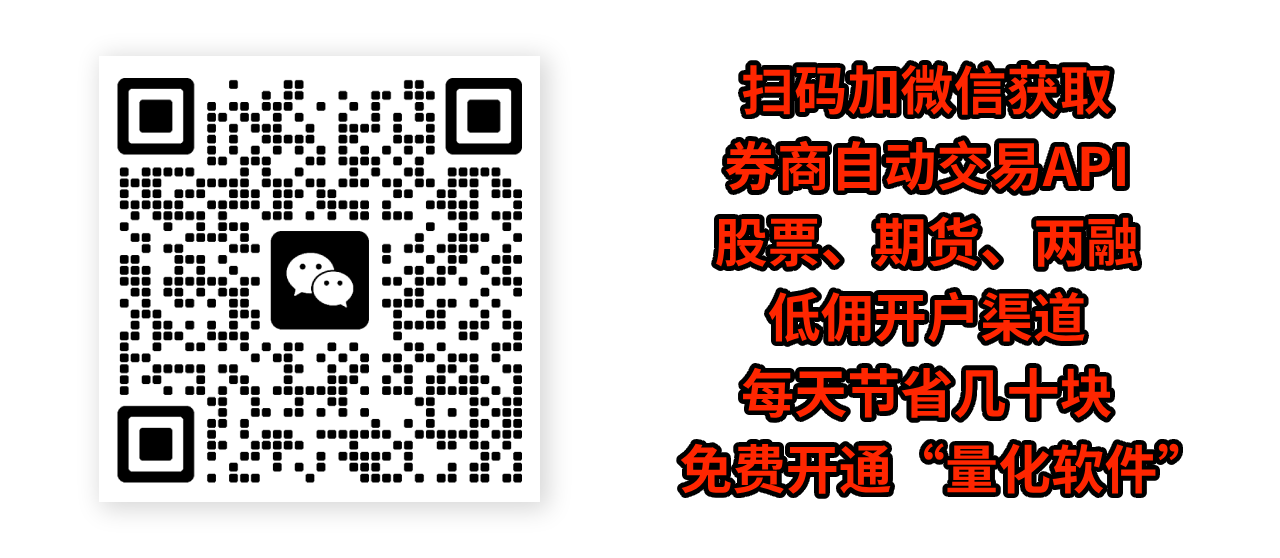
一起探讨:名词“独特外汇方案”的定义与作用
« 上一篇
2024-10-29
从零开始认识名词“独特外汇服务”
下一篇 »
2024-10-29