Python自动化炒股:基于自然语言处理的股票新闻情感分析模型开发与优化的最佳实践
Python自动化炒股:基于自然语言处理的股票新闻情感分析模型开发与优化的最佳实践
在当今的金融市场中,信息的快速流动和处理能力对投资者来说至关重要。Python作为一种强大的编程语言,结合自然语言处理(NLP)技术,可以帮助我们自动化分析股票新闻的情感倾向,从而为投资决策提供支持。本文将介绍如何开发和优化一个基于NLP的股票新闻情感分析模型。
1. 理解情感分析
情感分析,也称为情感挖掘,是指使用NLP技术来识别和提取文本中的主观信息。在股票新闻分析中,我们关注的是新闻报道对市场情绪的影响,正面新闻可能推动股价上涨,而负面新闻可能导致股价下跌。
2. 数据收集
首先,我们需要收集股票新闻数据。这可以通过网络爬虫实现,例如使用Python的requests
和BeautifulSoup
库。
import requests
from bs4 import BeautifulSoup
def fetch_news(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
news = soup.find_all('div', class_='news-content')
return [news_item.text for news_item in news]
# 示例URL
news_url = 'http://example.com/stock-news'
news_data = fetch_news(news_url)
3. 数据预处理
收集到的新闻数据需要进行预处理,包括去除停用词、标点符号、进行词干提取等。
import nltk
from nltk.corpus import stopwords
from nltk.stem import PorterStemmer
nltk.download('stopwords')
nltk.download('punkt')
stop_words = set(stopwords.words('english'))
stemmer = PorterStemmer()
def preprocess(text):
tokens = nltk.word_tokenize(text)
filtered_tokens = [stemmer.stem(word.lower()) for word in tokens if word.isalpha() and word.lower() not in stop_words]
return ' '.join(filtered_tokens)
processed_news = [preprocess(news) for news in news_data]
4. 情感分析模型
我们可以使用机器学习库如scikit-learn
来构建情感分析模型。这里以逻辑回归为例。
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import trAIn_test_split
from sklearn.metrics import accuracy_score
# 假设我们已经有了情感标签
labels = [1 if 'positive' in news else 0 for news in news_data] # 简化示例
X_train, X_test, y_train, y_test = train_test_split(processed_news, labels, test_size=0.2, random_state=42)
vectorizer = TfidfVectorizer()
X_train_vectors = vectorizer.fit_transform(X_train)
X_test_vectors = vectorizer.transform(X_test)
model = LogisticRegression()
model.fit(X_train_vectors, y_train)
predictions = model.predict(X_test_vectors)
print(f"Accuracy: {accuracy_score(y_test, predictions)}")
5. 模型优化
为了提高模型的准确性,我们可以尝试不同的特征提取方法、模型参数调整、集成学习等技术。
from sklearn.model_selection import GridSearchCV
# 参数网格
param_grid = {
'C': [0.1, 1, 10],
'penalty': ['l1', 'l2']
}
grid_search = GridSearchCV(LogisticRegression(), param_grid, cv=5)
grid_search.fit(X_train_vectors, y_train)
best_model = grid_search.best_estimator_
predictions = best_model.predict(X_test_vectors)
print(f"Optimized Accuracy: {accuracy_score(y_test, predictions)}")
6. 实时新闻分析
将模型部署为实时分析工具,可以监控新闻流并即时给出情感预测。
def analyze_realtime_news(news):
processed_news = preprocess(news)
news_vector = vectorizer.transform([processed_news])
prediction = best_model.predict(news_vector)
return 'Positive' if prediction[0] == 1 else 'Negative'
# 示例实时新闻
realtime_news = "Stock prices are expected to rise due to positive economic indicators."
print(analyze_realtime_news(realtime_news))
7. 结论
通过上述步骤,我们开发了一个基于NLP的股票新闻情感分析模型,并进行了优化。这个模型可以帮助投资者快速理解市场情绪,辅助投资决策。然而,需要注意的是,股市有风险,投资需谨慎,任何模型都不能保证100%的准确性。
8. 进一步探索
- 深度学习模型:尝试使用深度学习框架如TensorFlow或PyTorch构建更复杂的模型。
- 多语言支持:扩展模型以支持多种语言的新闻分析。
- 实时数据流:集成实时数据流服务,
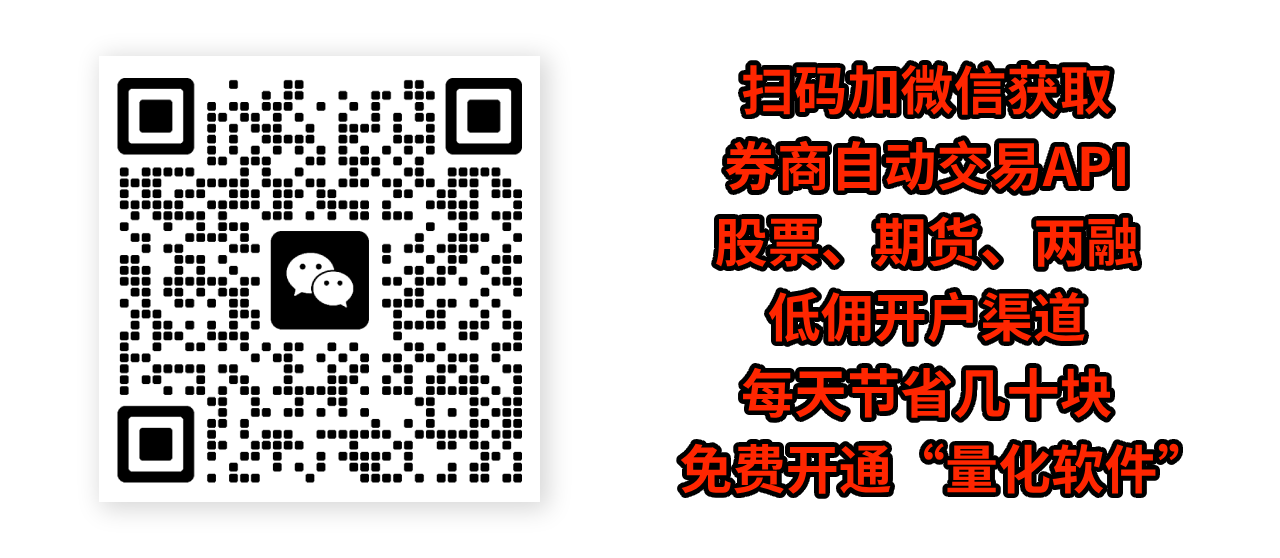
8.7 神经网络在量化投资中的应用
« 上一篇
2025-01-23
8.8 支持向量机在量化投资中的应用
下一篇 »
2025-01-25