Python自动化炒股:基于自然语言处理的股票新闻情感分析模型开发与优化的详细指南
Python自动化炒股:基于自然语言处理的股票新闻情感分析模型开发与优化的详细指南
在当今的金融市场中,信息的快速流通对于投资者来说至关重要。股票新闻作为市场情绪的晴雨表,其情感倾向往往能影响投资者的决策。本文将带你深入了解如何使用Python和自然语言处理(NLP)技术来开发一个股票新闻情感分析模型,以辅助自动化炒股决策。
1. 理解情感分析
情感分析,又称为情感挖掘,是指使用NLP技术来识别和提取文本中的主观信息,如情绪、意见、评价等。在股票新闻分析中,我们关注的是新闻文本对市场情绪的影响,是正面还是负面。
2. 数据收集
首先,我们需要收集股票新闻数据。这可以通过网络爬虫实现,例如使用requests
和BeautifulSoup
库。
import requests
from bs4 import BeautifulSoup
def fetch_news(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
news = soup.find_all('div', class_='news-content')
return [news_item.text for news_item in news]
# 示例URL,实际使用时请替换为有效的新闻网站URL
news_urls = ['http://example.com/news1', 'http://example.com/news2']
news_data = [fetch_news(url) for url in news_urls]
3. 数据预处理
收集到的新闻数据需要进行预处理,包括去除停用词、标点符号、进行词干提取等。
import nltk
from nltk.corpus import stopwords
from nltk.stem import PorterStemmer
nltk.download('stopwords')
nltk.download('punkt')
stop_words = set(stopwords.words('english'))
stemmer = PorterStemmer()
def preprocess(text):
tokens = nltk.word_tokenize(text)
filtered_tokens = [stemmer.stem(word) for word in tokens if word not in stop_words and word.isalpha()]
return ' '.join(filtered_tokens)
processed_news = [preprocess(news) for news in news_data]
4. 情感分析模型
我们将使用机器学习库scikit-learn
来构建一个简单的情感分析模型。这里以逻辑回归为例。
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.model_selection import trAIn_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import classification_report
# 假设我们已经有了标签数据
labels = [1, 0, 1, 1, 0] # 1代表正面,0代表负面
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(processed_news)
y = labels
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = LogisticRegression()
model.fit(X_train, y_train)
predictions = model.predict(X_test)
print(classification_report(y_test, predictions))
5. 模型优化
为了提高模型的准确性,我们可以尝试不同的特征提取方法,如TF-IDF,或者使用更复杂的模型,如支持向量机(SVM)或深度学习模型。
from sklearn.feature_extraction.text import TfidfVectorizer
tfidf_vectorizer = TfidfVectorizer()
X_tfidf = tfidf_vectorizer.fit_transform(processed_news)
# 使用TF-IDF特征重新训练模型
model.fit(X_tfidf_train, y_train)
predictions_tfidf = model.predict(X_tfidf_test)
print(classification_report(y_test, predictions_tfidf))
6. 集成学习
集成学习是一种提高模型性能的有效方法,通过组合多个模型的预测来提高整体的准确性。
from sklearn.ensemble import RandomForestClassifier
rf_model = RandomForestClassifier()
rf_model.fit(X_train, y_train)
predictions_rf = rf_model.predict(X_test)
print(classification_report(y_test, predictions_rf))
7. 实时新闻分析
将模型部署为一个实时新闻分析系统,可以监控新闻网站,自动分析新闻情感,并给出投资建议。
import time
def real_time_analysis(model, vectorizer):
while True:
news = fetch_news('http://example.com/news')
processed_news = preprocess(news)
vectorized_news = vectorizer.transform([processed_news])
prediction = model.predict(vectorized_news)
print(f"News sentiment: {'Positive' if prediction[0] == 1 else 'Negative'}")
time.sleep(3600) # 每小时检查一次
real_time_analysis(model, vectorizer)
8. 结论
通过本文的指南,你已经学会了如何使用Python和NLP技术来开发一个股票新闻情感分析模型。这个模型可以帮助
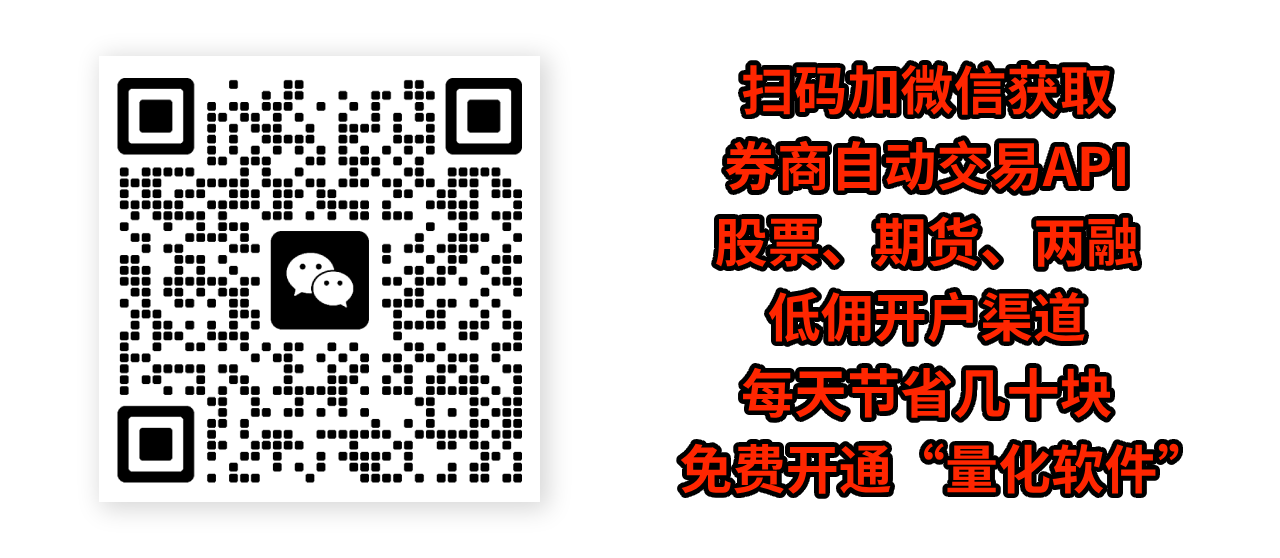
了解名词“短线趋势咨询”:从基础到深入
« 上一篇
2024-12-10
7.16 特征工程在量化投资中的应用
下一篇 »
2024-12-10