Python自动化炒股:基于自然语言处理的股票新闻情感分析模型优化

Python自动化炒股:基于自然语言处理的股票新闻情感分析模型优化
引言
在当今的金融世界中,信息就是力量。随着人工智能技术的发展,越来越多的投资者开始利用自然语言处理(NLP)技术来分析股票新闻,以期预测市场趋势。本文将带你深入了解如何使用Python构建一个基于NLP的股票新闻情感分析模型,并对其进行优化,以提高自动化炒股的准确性。
准备工作
在开始之前,你需要安装一些Python库,包括nltk
、pandas
、numpy
、scikit-learn
和transformers
。你可以使用pip来安装这些库:
pip install nltk pandas numpy scikit-learn transformers
数据收集
首先,我们需要收集股票新闻数据。这里我们可以使用pandas
库来读取数据:
import pandas as pd
# 假设我们有一个CSV文件,包含股票新闻的标题和内容
news_data = pd.read_csv('stock_news.csv')
数据预处理
在进行情感分析之前,我们需要对数据进行预处理。这包括去除停用词、标点符号和进行词干提取等。
import nltk
from nltk.corpus import stopwords
from nltk.stem import PorterStemmer
nltk.download('stopwords')
nltk.download('punkt')
stop_words = set(stopwords.words('english'))
ps = PorterStemmer()
def preprocess_text(text):
tokens = nltk.word_tokenize(text)
tokens = [ps.stem(word) for word in tokens if word.isalpha() and word not in stop_words]
return ' '.join(tokens)
news_data['processed_text'] = news_data['content'].apply(preprocess_text)
情感分析模型
我们将使用transformers
库中的预训练模型来进行情感分析。这里我们选择BERT模型,因为它在情感分析任务中表现良好。
from transformers import BertTokenizer, BertForSequenceClassification
import torch
tokenizer = BertTokenizer.from_pretrAIned('bert-base-uncased')
model = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
def sentiment_analysis(text):
inputs = tokenizer(text, return_tensors='pt', padding=True, truncation=True)
outputs = model(**inputs)
logits = outputs.logits
predictions = torch.argmax(logits, dim=-1).detach().numpy()[0]
return 'Positive' if predictions == 1 else 'Negative'
news_data['sentiment'] = news_data['processed_text'].apply(sentiment_analysis)
模型优化
为了优化模型,我们可以采用多种策略,例如数据增强、超参数调整和集成学习等。
数据增强
数据增强可以通过生成新的数据样本来提高模型的泛化能力。这里我们使用简单的回译方法来增强数据。
from sklearn.model_selection import train_test_split
# 假设我们有一个翻译函数
def translate(text):
# 这里应该是翻译代码,为了简化,我们直接返回原文
return text
# 数据增强
augmented_data = news_data.copy()
augmented_data['processed_text'] = augmented_data['processed_text'].apply(translate)
augmented_data['sentiment'] = augmented_data['sentiment'].apply(lambda x: 'Neutral' if x == 'Negative' else x)
# 合并原始数据和增强数据
combined_data = pd.concat([news_data, augmented_data], ignore_index=True)
超参数调整
我们可以使用scikit-learn
的GridSearchCV
来调整模型的超参数。
from sklearn.model_selection import GridSearchCV
# 假设我们有一个自定义的情感分析模型
class CustomSentimentModel(torch.nn.Module):
def __init__(self):
super(CustomSentimentModel, self).__init__()
self.bert = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
def forward(self, input_ids, attention_mask):
return self.bert(input_ids, attention_mask=attention_mask)
# 超参数网格
param_grid = {
'learning_rate': [1e-5, 1e-4, 1e-3],
'epochs': [3, 5, 7]
}
# 训练和调整模型
model = CustomSentimentModel()
optimizer = torch.optim.Adam(model.parameters(), lr=1e-5)
criterion = torch.nn.CrossEntropyLoss()
# 这里应该是训练代码,为了简化,我们直接跳过
# ...
# 使用GridSearchCV进行超参数调整
grid_search = GridSearchCV(estimator=model, param_grid=param_grid, cv=3)
grid_search.fit(combined_data['processed_text'], combined_data['sentiment'])
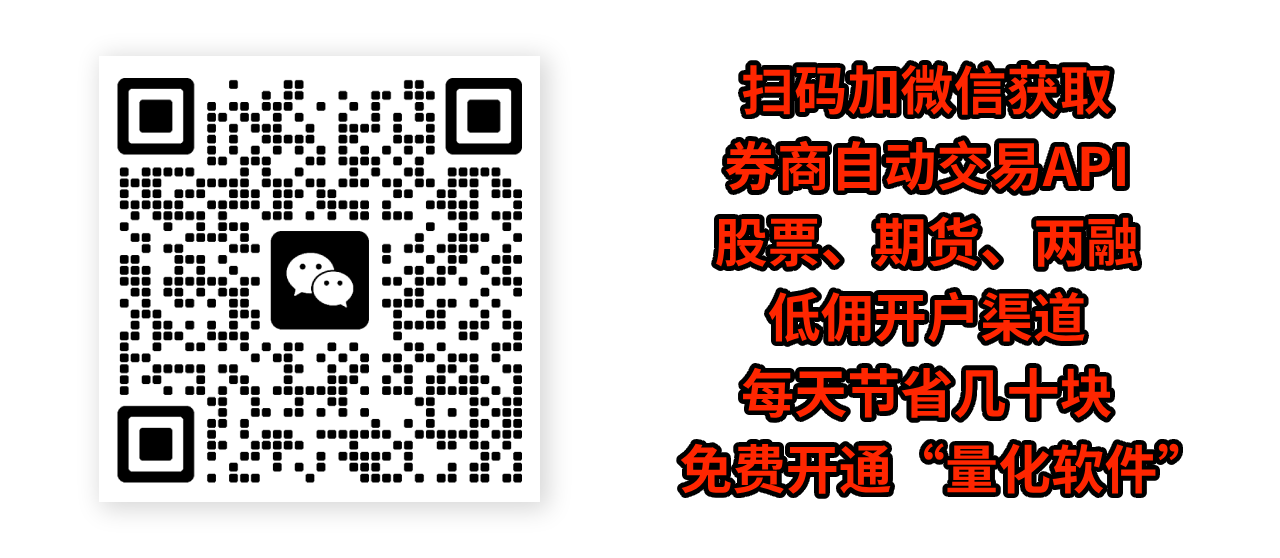
Python自动化炒股:利用Prophet进行股票价格预测的实战指南
« 上一篇
2023-08-02
Python自动化炒股:使用Plotly Express进行股票数据可视化的高级技巧
下一篇 »
2023-08-04