Python自动化炒股:基于强化学习的股票交易策略优化与实现的实战案例
Python自动化炒股:基于强化学习的股票交易策略优化与实现的实战案例
引言
在金融领域,自动化交易策略一直是投资者和交易员追求的目标。随着机器学习和人工智能技术的发展,强化学习(Reinforcement Learning, RL)作为一种决策框架,被越来越多的应用于股票交易策略的优化。本文将介绍如何使用Python实现基于强化学习的股票交易策略,并提供一个实战案例。
强化学习基础
强化学习是一种无模型的学习方法,通过与环境的交互来学习最优策略。在股票交易中,环境可以是股票市场,而智能体(Agent)则是我们的交易策略。智能体通过执行动作(如买入、卖出)来获得奖励(如利润),并根据奖励来更新策略。
环境(Environment)
在股票交易中,环境可以定义为股票市场,它提供了股票价格、交易量等信息。智能体需要根据这些信息来做出决策。
状态(State)
状态是智能体观察到的环境信息,可以包括股票价格、交易量、历史价格变化等。
动作(Action)
动作是智能体可以执行的操作,例如买入、卖出或持有。
奖励(Reward)
奖励是智能体执行动作后获得的反馈,通常与利润相关。
Python实现
我们将使用Python的gym
库来模拟股票交易环境,并使用stable-baselines3
库来实现强化学习算法。
安装必要的库
!pip install gym stable-baselines3 pandas numpy
定义股票交易环境
import gym
from gym import spaces
import numpy as np
import pandas as pd
class StockTradingEnv(gym.Env):
metadata = {'render.modes': ['console']}
def __init__(self, df, initial_balance=1000.0, buy_cost_pct=0.001, sell_cost_pct=0.001, reward_scaling=1e-4):
self.df = df
self.balance = initial_balance
self.buy_cost_pct = buy_cost_pct
self.sell_cost_pct = sell_cost_pct
self.reward_scaling = reward_scaling
self.shares = 0
self.state = None
self.action_space = spaces.Box(low=-1, high=1, shape=(1,), dtype=np.float32) # -1 sell, 0 hold, 1 buy
self.observation_space = spaces.Box(low=-np.inf, high=np.inf, shape=(6,), dtype=np.float32)
def reset(self):
self.balance = 1000.0
self.shares = 0
self.state = self.df.iloc[0].values
return self.state
def step(self, action):
# Implement the logic for one time step
pass
def render(self, mode='console'):
# Implement the rendering logic
pass
训练强化学习模型
我们将使用stable-baselines3
库中的A2C
算法来训练我们的模型。
from stable_baselines3 import A2C
from stable_baselines3.common.env_util import make_vec_env
# 假设df是包含股票历史数据的Pandas DataFrame
env = StockTradingEnv(df)
env = make_vec_env(lambda: env, n_envs=1)
model = A2C('MlpPolicy', env, verbose=1)
model.learn(total_timesteps=10000)
# 保存模型
model.save("stock_trading_model")
测试模型
# 加载模型
model = A2C.load("stock_trading_model", env=env)
# 测试模型
obs = env.reset()
done = False
while not done:
action, _states = model.predict(obs)
obs, rewards, done, info = env.step(action)
env.render()
实战案例
假设我们有一支股票的历史价格数据,我们将使用上述模型来测试其性能。
数据准备
# 假设df是包含股票历史数据的Pandas DataFrame
# 这里我们使用随机数据作为示例
import random
dates = pd.date_range('20230101', periods=100)
prices = [random.randint(100, 200) for _ in range(100)]
df = pd.DataFrame({'Date': dates, 'Price': prices})
训练和测试
按照上述步骤,我们可以训练模型并在测试集上评估其性能。
结论
通过使用Python和强化学习,我们可以构建自动化的股票交易策略。这种方法可以帮助我们优化交易决策,提高投资回报。然而,需要注意的是,股票市场具有高度不确定性,任何
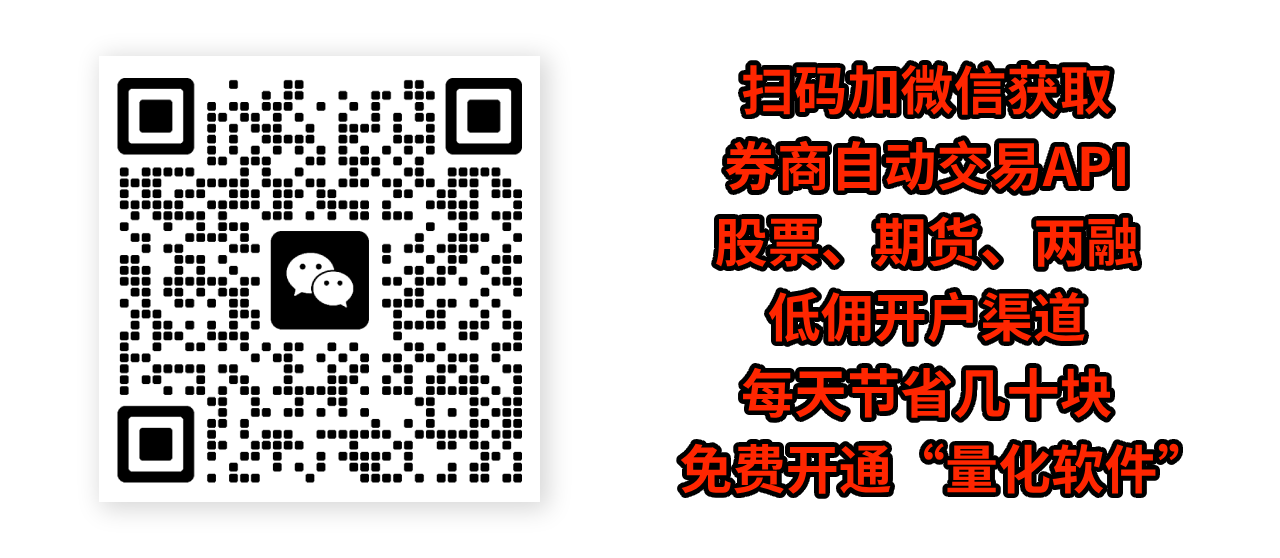
一起探讨:名词“优选成交预测”的定义与作用
« 上一篇
2023-11-04
名词“优选债券管理”的核心概念及实际意义
下一篇 »
2023-11-04