Python自动化炒股:基于强化学习的股票交易策略优化与实现的详细指南
Python自动化炒股:基于强化学习的股票交易策略优化与实现的详细指南
在金融市场中,自动化交易策略因其高效性和客观性而受到投资者的青睐。近年来,强化学习(Reinforcement Learning, RL)作为一种先进的机器学习方法,被广泛应用于股票交易策略的优化。本文将带你深入了解如何使用Python实现基于强化学习的股票交易策略,并提供详细的代码示例。
强化学习简介
强化学习是一种让智能体(agent)通过与环境(environment)的交互来学习最优策略的方法。在股票交易中,智能体的目标是最大化其累积奖励,即投资回报。环境则提供股票价格、交易成本等信息,智能体根据这些信息做出买卖决策。
环境设置
首先,我们需要创建一个模拟股票交易的环境。这里我们使用gym
库来构建环境。
import gym
from gym import spaces
import numpy as np
class StockTradingEnv(gym.Env):
metadata = {'render.modes': ['human']}
def __init__(self, initial_balance=1000, transaction_cost=0.001):
super(StockTradingEnv, self).__init__()
self.initial_balance = initial_balance
self.transaction_cost = transaction_cost
self.balance = initial_balance
self.shares = 0
self.action_space = spaces.Box(low=-1, high=1, shape=(1,), dtype=np.float32)
self.observation_space = spaces.Box(low=0, high=np.inf, shape=(2,), dtype=np.float32)
def reset(self):
self.balance = self.initial_balance
self.shares = 0
return np.array([self.balance, self.shares])
def step(self, action):
# 这里简化了交易逻辑,实际应用中需要更复杂的处理
self.shares = action[0] * self.balance
self.balance -= self.shares * self.transaction_cost
reward = self.shares * (1 + np.random.normal(0, 0.01)) - self.transaction_cost * self.shares
done = False
return np.array([self.balance, self.shares]), reward, done, {}
def render(self, mode='human', close=False):
if close:
return
print(f'Balance: {self.balance}, Shares: {self.shares}')
# 创建环境实例
env = StockTradingEnv()
策略实现
接下来,我们使用DQN(Deep Q-Network)算法来实现交易策略。DQN是一种结合了深度学习和Q学习的强化学习算法。
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
class DQNAgent:
def __init__(self, state_size, action_size):
self.state_size = state_size
self.action_size = action_size
self.memory = []
self.gamma = 0.95 # discount rate
self.epsilon = 1.0 # exploration rate
self.epsilon_min = 0.01
self.epsilon_decay = 0.995
self.learning_rate = 0.001
self.model = self._build_model()
def _build_model(self):
model = Sequential()
model.add(Dense(24, input_dim=self.state_size, activation='relu'))
model.add(Dense(24, activation='relu'))
model.add(Dense(self.action_size, activation='linear'))
model.compile(loss='mse', optimizer=tf.keras.optimizers.Adam(lr=self.learning_rate))
return model
def remember(self, state, action, reward, next_state, done):
self.memory.append((state, action, reward, next_state, done))
def act(self, state):
if np.random.rand() <= self.epsilon:
return np.random.choice(self.action_size)
act_values = self.model.predict(state)
return np.argmax(act_values[0])
def replay(self, batch_size):
minibatch = random.sample(self.memory, batch_size)
for state, action, reward, next_state, done in minibatch:
target = reward
if not done:
target = (reward + self.gamma * np.amax(self.model.predict(next_state)[0]))
target_f = self.model.predict(state)
target_f[0][action] = target
self.model.fit(state, target_f, epochs=1, verbose=0)
if self.epsilon > self.epsilon_min:
self.epsilon *= self.epsilon_decay
训练与测试
现在,我们可以训练我们的DQN代理,并在模拟环境中测试其性能。
# 初始化代理
agent = DQNAgent(state_size=2, action_size=1)
# 训练过程
episodes = 1000
batch_size = 32
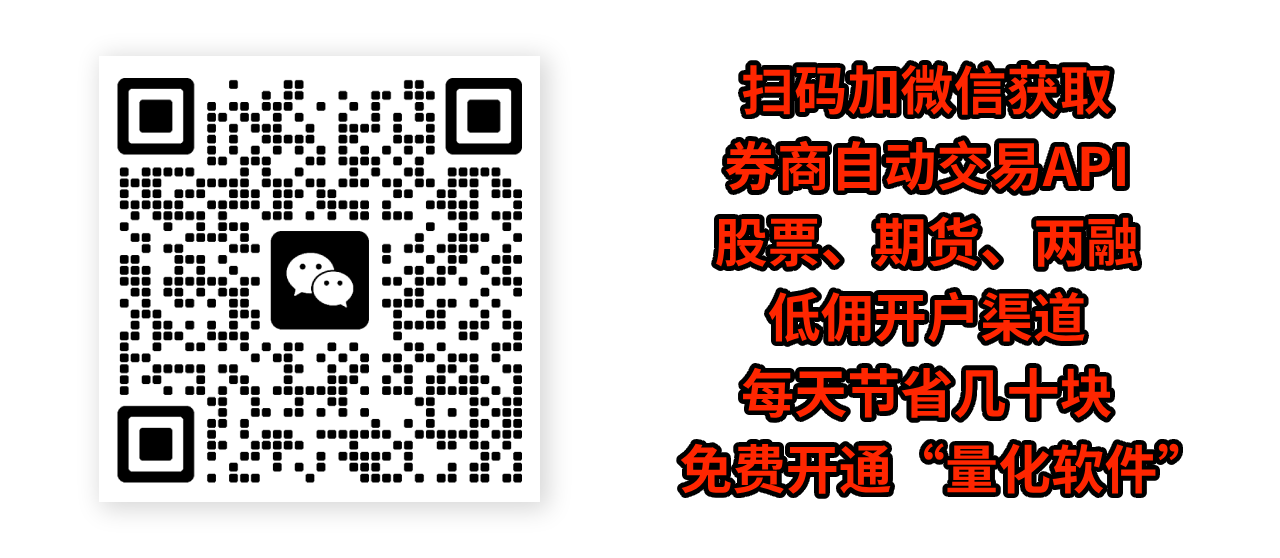
Python自动化炒股:利用XGBoost和LightGBM进行股票市场预测的实战案例
« 上一篇
2023-09-04
Python自动化炒股:使用FastAPI和Kubernetes部署股票数据服务的最佳实践
下一篇 »
2023-09-06