Python自动化炒股:基于自然语言处理的股票新闻情感分析模型开发与优化的最佳实践
Python自动化炒股:基于自然语言处理的股票新闻情感分析模型开发与优化的最佳实践
在当今的金融市场中,信息流动的速度和质量对投资决策的影响越来越大。自然语言处理(NLP)技术的发展为投资者提供了一种新的工具,通过分析股票新闻的情感倾向来预测市场动向。本文将介绍如何使用Python开发一个基于NLP的股票新闻情感分析模型,并探讨模型优化的最佳实践。
1. 理解情感分析
情感分析,又称为情感挖掘,是指使用NLP技术来识别和提取文本中的主观信息,如情绪、情感倾向等。在股票新闻分析中,我们关注的是新闻文本中的情感倾向,如正面、负面或中性。
2. 数据收集
首先,我们需要收集股票新闻数据。这里我们可以使用Python的requests
库来从网络爬取数据。
import requests
from bs4 import BeautifulSoup
def fetch_news(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
news = soup.find_all('div', class_='news-content')
return [n.text for n in news]
# 示例URL,实际应用中需要替换为有效的新闻源URL
news_data = fetch_news('https://example.com/stock-news')
3. 数据预处理
收集到的新闻数据需要进行预处理,包括去除停用词、标点符号等。
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
nltk.download('punkt')
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
def preprocess_text(text):
tokens = word_tokenize(text)
filtered_text = [word for word in tokens if word not in stop_words and word.isalpha()]
return " ".join(filtered_text)
processed_news = [preprocess_text(news) for news in news_data]
4. 情感分析模型开发
我们可以使用Python的TextBlob
库来开发一个简单的情感分析模型。
from textblob import TextBlob
def analyze_sentiment(text):
blob = TextBlob(text)
return blob.sentiment.polarity
sentiments = [analyze_sentiment(news) for news in processed_news]
5. 模型评估
为了评估模型的效果,我们可以计算准确率、召回率等指标。
from sklearn.metrics import accuracy_score, recall_score
# 假设我们有真实的情感标签
true_sentiments = [1, 0, 1, 0, 1] # 1代表正面,0代表负面
# 将情感倾向转换为二分类标签
predicted_labels = [1 if sentiment > 0 else 0 for sentiment in sentiments]
accuracy = accuracy_score(true_sentiments, predicted_labels)
recall = recall_score(true_sentiments, predicted_labels)
print(f'Accuracy: {accuracy}')
print(f'Recall: {recall}')
6. 模型优化
模型优化是一个持续的过程,我们可以通过以下方法来优化模型:
- 特征工程:提取更有意义的特征,如TF-IDF、Word2Vec等。
- 模型选择:尝试不同的模型,如逻辑回归、SVM、深度学习模型等。
- 超参数调优:使用网格搜索等方法来找到最优的模型参数。
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.svm import SVC
from sklearn.model_selection import GridSearchCV
# 特征提取
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(processed_news)
# 模型训练
clf = SVC()
parameters = {'C': [0.1, 1, 10], 'kernel': ['linear', 'rbf']}
grid_search = GridSearchCV(clf, parameters, cv=5)
grid_search.fit(X, true_sentiments)
# 最优参数
print(grid_search.best_params_)
7. 实时监控与反馈
在实际应用中,我们需要实时监控模型的表现,并根据反馈进行调整。
def monitor_model(model, new_data):
new_predictions = model.predict(new_data)
# 这里可以添加代码来评估新预测的表现,并根据需要调整模型
pass
# 假设new_news_data是新收集的新闻数据
new_news_data = fetch_news('https://example.com/new-stock-news')
new_processed_news = [preprocess_text(news) for news in new_news_data]
monitor_model(grid_search.best_estimator_, new_processed_news)
8. 结论
通过本文的介绍,我们了解了如何使用Python开发一个基于自然语言处理的股票新闻情感分析模型,并探讨了模型优化的最佳实践。情感分析模型的开发和优化是一个复杂的过程,需要不断地实验和调整。希望本文能为你在自动化
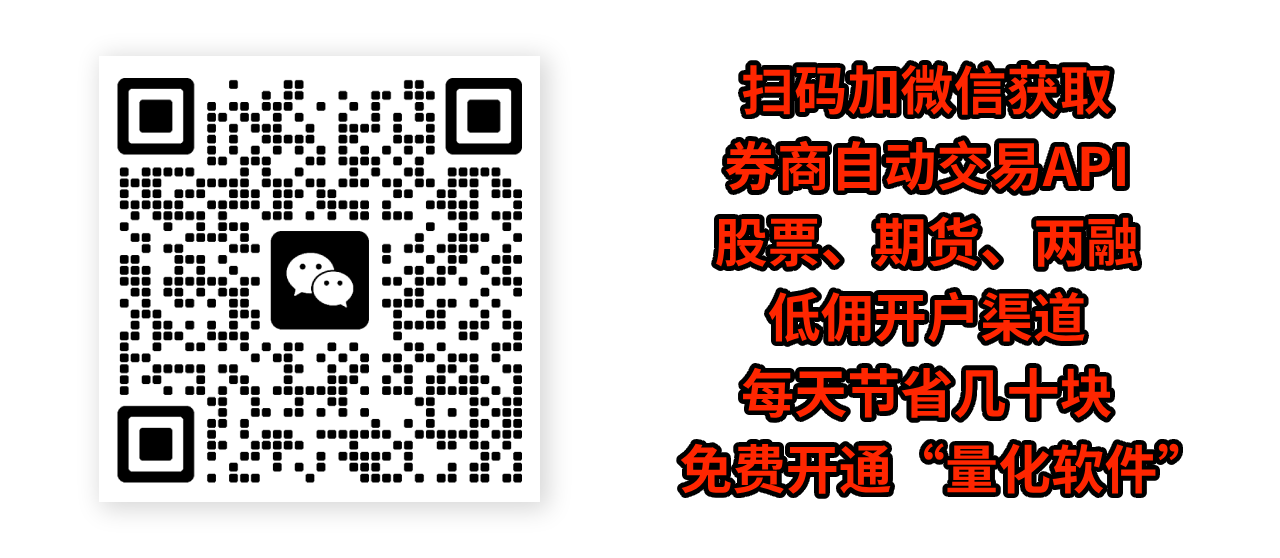
剖析名词“优质估值管理”:背后的概念
« 上一篇
2023-10-17
【解析】名词“优质可转债报告”的内涵与外延
下一篇 »
2023-10-17